MDS#
- class sklearn.manifold.MDS(n_components=2, *, metric=True, n_init=4, max_iter=300, verbose=0, eps=0.001, n_jobs=None, random_state=None, dissimilarity='euclidean', normalized_stress='auto')[source]#
Multidimensional scaling.
Read more in the User Guide.
- Parameters:
- n_componentsint, default=2
Number of dimensions in which to immerse the dissimilarities.
- metricbool, default=True
If
True
, perform metric MDS; otherwise, perform nonmetric MDS. WhenFalse
(i.e. non-metric MDS), dissimilarities with 0 are considered as missing values.- n_initint, default=4
Number of times the SMACOF algorithm will be run with different initializations. The final results will be the best output of the runs, determined by the run with the smallest final stress.
- max_iterint, default=300
Maximum number of iterations of the SMACOF algorithm for a single run.
- verboseint, default=0
Level of verbosity.
- epsfloat, default=1e-3
Relative tolerance with respect to stress at which to declare convergence. The value of
eps
should be tuned separately depending on whether or notnormalized_stress
is being used.- n_jobsint, default=None
The number of jobs to use for the computation. If multiple initializations are used (
n_init
), each run of the algorithm is computed in parallel.None
means 1 unless in ajoblib.parallel_backend
context.-1
means using all processors. See Glossary for more details.- random_stateint, RandomState instance or None, default=None
Determines the random number generator used to initialize the centers. Pass an int for reproducible results across multiple function calls. See Glossary.
- dissimilarity{‘euclidean’, ‘precomputed’}, default=’euclidean’
Dissimilarity measure to use:
- ‘euclidean’:
Pairwise Euclidean distances between points in the dataset.
- ‘precomputed’:
Pre-computed dissimilarities are passed directly to
fit
andfit_transform
.
- normalized_stressbool or “auto” default=”auto”
Whether use and return normed stress value (Stress-1) instead of raw stress calculated by default. Only supported in non-metric MDS.
Added in version 1.2.
Changed in version 1.4: The default value changed from
False
to"auto"
in version 1.4.
- Attributes:
- embedding_ndarray of shape (n_samples, n_components)
Stores the position of the dataset in the embedding space.
- stress_float
The final value of the stress (sum of squared distance of the disparities and the distances for all constrained points). If
normalized_stress=True
, andmetric=False
returns Stress-1. A value of 0 indicates “perfect” fit, 0.025 excellent, 0.05 good, 0.1 fair, and 0.2 poor [1].- dissimilarity_matrix_ndarray of shape (n_samples, n_samples)
Pairwise dissimilarities between the points. Symmetric matrix that:
either uses a custom dissimilarity matrix by setting
dissimilarity
to ‘precomputed’;or constructs a dissimilarity matrix from data using Euclidean distances.
- n_features_in_int
Number of features seen during fit.
Added in version 0.24.
- feature_names_in_ndarray of shape (
n_features_in_
,) Names of features seen during fit. Defined only when
X
has feature names that are all strings.Added in version 1.0.
- n_iter_int
The number of iterations corresponding to the best stress.
See also
sklearn.decomposition.PCA
Principal component analysis that is a linear dimensionality reduction method.
sklearn.decomposition.KernelPCA
Non-linear dimensionality reduction using kernels and PCA.
TSNE
T-distributed Stochastic Neighbor Embedding.
Isomap
Manifold learning based on Isometric Mapping.
LocallyLinearEmbedding
Manifold learning using Locally Linear Embedding.
SpectralEmbedding
Spectral embedding for non-linear dimensionality.
References
[1]“Nonmetric multidimensional scaling: a numerical method” Kruskal, J. Psychometrika, 29 (1964)
[2]“Multidimensional scaling by optimizing goodness of fit to a nonmetric hypothesis” Kruskal, J. Psychometrika, 29, (1964)
[3]“Modern Multidimensional Scaling - Theory and Applications” Borg, I.; Groenen P. Springer Series in Statistics (1997)
Examples
>>> from sklearn.datasets import load_digits >>> from sklearn.manifold import MDS >>> X, _ = load_digits(return_X_y=True) >>> X.shape (1797, 64) >>> embedding = MDS(n_components=2, normalized_stress='auto') >>> X_transformed = embedding.fit_transform(X[:100]) >>> X_transformed.shape (100, 2)
For a more detailed example of usage, see Multi-dimensional scaling.
For a comparison of manifold learning techniques, see Comparison of Manifold Learning methods.
- fit(X, y=None, init=None)[source]#
Compute the position of the points in the embedding space.
- Parameters:
- Xarray-like of shape (n_samples, n_features) or (n_samples, n_samples)
Input data. If
dissimilarity=='precomputed'
, the input should be the dissimilarity matrix.- yIgnored
Not used, present for API consistency by convention.
- initndarray of shape (n_samples, n_components), default=None
Starting configuration of the embedding to initialize the SMACOF algorithm. By default, the algorithm is initialized with a randomly chosen array.
- Returns:
- selfobject
Fitted estimator.
- fit_transform(X, y=None, init=None)[source]#
Fit the data from
X
, and returns the embedded coordinates.- Parameters:
- Xarray-like of shape (n_samples, n_features) or (n_samples, n_samples)
Input data. If
dissimilarity=='precomputed'
, the input should be the dissimilarity matrix.- yIgnored
Not used, present for API consistency by convention.
- initndarray of shape (n_samples, n_components), default=None
Starting configuration of the embedding to initialize the SMACOF algorithm. By default, the algorithm is initialized with a randomly chosen array.
- Returns:
- X_newndarray of shape (n_samples, n_components)
X transformed in the new space.
- get_metadata_routing()[source]#
Get metadata routing of this object.
Please check User Guide on how the routing mechanism works.
- Returns:
- routingMetadataRequest
A
MetadataRequest
encapsulating routing information.
- get_params(deep=True)[source]#
Get parameters for this estimator.
- Parameters:
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns:
- paramsdict
Parameter names mapped to their values.
- set_fit_request(*, init: bool | None | str = '$UNCHANGED$') MDS [source]#
Request metadata passed to the
fit
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed tofit
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it tofit
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.Added in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- initstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
init
parameter infit
.
- Returns:
- selfobject
The updated object.
- set_params(**params)[source]#
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters:
- **paramsdict
Estimator parameters.
- Returns:
- selfestimator instance
Estimator instance.
Gallery examples#
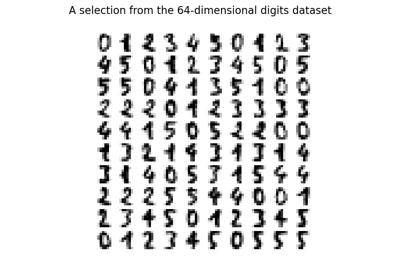
Manifold learning on handwritten digits: Locally Linear Embedding, Isomap…