sklearn.calibration
.CalibratedClassifierCV¶
- class sklearn.calibration.CalibratedClassifierCV(estimator=None, *, method='sigmoid', cv=None, n_jobs=None, ensemble=True)[source]¶
Probability calibration with isotonic regression or logistic regression.
This class uses cross-validation to both estimate the parameters of a classifier and subsequently calibrate a classifier. With default
ensemble=True
, for each cv split it fits a copy of the base estimator to the training subset, and calibrates it using the testing subset. For prediction, predicted probabilities are averaged across these individual calibrated classifiers. Whenensemble=False
, cross-validation is used to obtain unbiased predictions, viacross_val_predict
, which are then used for calibration. For prediction, the base estimator, trained using all the data, is used. This is the prediction method implemented whenprobabilities=True
forSVC
andNuSVC
estimators (see User Guide for details).Already fitted classifiers can be calibrated via the parameter
cv="prefit"
. In this case, no cross-validation is used and all provided data is used for calibration. The user has to take care manually that data for model fitting and calibration are disjoint.The calibration is based on the decision_function method of the
estimator
if it exists, else on predict_proba.Read more in the User Guide.
- Parameters:
- estimatorestimator instance, default=None
The classifier whose output need to be calibrated to provide more accurate
predict_proba
outputs. The default classifier is aLinearSVC
.New in version 1.2.
- method{‘sigmoid’, ‘isotonic’}, default=’sigmoid’
The method to use for calibration. Can be ‘sigmoid’ which corresponds to Platt’s method (i.e. a logistic regression model) or ‘isotonic’ which is a non-parametric approach. It is not advised to use isotonic calibration with too few calibration samples
(<<1000)
since it tends to overfit.- cvint, cross-validation generator, iterable or “prefit”, default=None
Determines the cross-validation splitting strategy. Possible inputs for cv are:
None, to use the default 5-fold cross-validation,
integer, to specify the number of folds.
An iterable yielding (train, test) splits as arrays of indices.
For integer/None inputs, if
y
is binary or multiclass,StratifiedKFold
is used. Ify
is neither binary nor multiclass,KFold
is used.Refer to the User Guide for the various cross-validation strategies that can be used here.
If “prefit” is passed, it is assumed that
estimator
has been fitted already and all data is used for calibration.Changed in version 0.22:
cv
default value if None changed from 3-fold to 5-fold.- n_jobsint, default=None
Number of jobs to run in parallel.
None
means 1 unless in ajoblib.parallel_backend
context.-1
means using all processors.Base estimator clones are fitted in parallel across cross-validation iterations. Therefore parallelism happens only when
cv != "prefit"
.See Glossary for more details.
New in version 0.24.
- ensemblebool, default=True
Determines how the calibrator is fitted when
cv
is not'prefit'
. Ignored ifcv='prefit'
.If
True
, theestimator
is fitted using training data, and calibrated using testing data, for eachcv
fold. The final estimator is an ensemble ofn_cv
fitted classifier and calibrator pairs, wheren_cv
is the number of cross-validation folds. The output is the average predicted probabilities of all pairs.If
False
,cv
is used to compute unbiased predictions, viacross_val_predict
, which are then used for calibration. At prediction time, the classifier used is theestimator
trained on all the data. Note that this method is also internally implemented insklearn.svm
estimators with theprobabilities=True
parameter.New in version 0.24.
- Attributes:
- classes_ndarray of shape (n_classes,)
The class labels.
- n_features_in_int
Number of features seen during fit. Only defined if the underlying estimator exposes such an attribute when fit.
New in version 0.24.
- feature_names_in_ndarray of shape (
n_features_in_
,) Names of features seen during fit. Only defined if the underlying estimator exposes such an attribute when fit.
New in version 1.0.
- calibrated_classifiers_list (len() equal to cv or 1 if
cv="prefit"
orensemble=False
) The list of classifier and calibrator pairs.
When
cv="prefit"
, the fittedestimator
and fitted calibrator.When
cv
is not “prefit” andensemble=True
,n_cv
fittedestimator
and calibrator pairs.n_cv
is the number of cross-validation folds.When
cv
is not “prefit” andensemble=False
, theestimator
, fitted on all the data, and fitted calibrator.
Changed in version 0.24: Single calibrated classifier case when
ensemble=False
.
See also
calibration_curve
Compute true and predicted probabilities for a calibration curve.
References
[1]Obtaining calibrated probability estimates from decision trees and naive Bayesian classifiers, B. Zadrozny & C. Elkan, ICML 2001
[2]Transforming Classifier Scores into Accurate Multiclass Probability Estimates, B. Zadrozny & C. Elkan, (KDD 2002)
[3]Probabilistic Outputs for Support Vector Machines and Comparisons to Regularized Likelihood Methods, J. Platt, (1999)
[4]Predicting Good Probabilities with Supervised Learning, A. Niculescu-Mizil & R. Caruana, ICML 2005
Examples
>>> from sklearn.datasets import make_classification >>> from sklearn.naive_bayes import GaussianNB >>> from sklearn.calibration import CalibratedClassifierCV >>> X, y = make_classification(n_samples=100, n_features=2, ... n_redundant=0, random_state=42) >>> base_clf = GaussianNB() >>> calibrated_clf = CalibratedClassifierCV(base_clf, cv=3) >>> calibrated_clf.fit(X, y) CalibratedClassifierCV(...) >>> len(calibrated_clf.calibrated_classifiers_) 3 >>> calibrated_clf.predict_proba(X)[:5, :] array([[0.110..., 0.889...], [0.072..., 0.927...], [0.928..., 0.071...], [0.928..., 0.071...], [0.071..., 0.928...]]) >>> from sklearn.model_selection import train_test_split >>> X, y = make_classification(n_samples=100, n_features=2, ... n_redundant=0, random_state=42) >>> X_train, X_calib, y_train, y_calib = train_test_split( ... X, y, random_state=42 ... ) >>> base_clf = GaussianNB() >>> base_clf.fit(X_train, y_train) GaussianNB() >>> calibrated_clf = CalibratedClassifierCV(base_clf, cv="prefit") >>> calibrated_clf.fit(X_calib, y_calib) CalibratedClassifierCV(...) >>> len(calibrated_clf.calibrated_classifiers_) 1 >>> calibrated_clf.predict_proba([[-0.5, 0.5]]) array([[0.936..., 0.063...]])
Methods
fit
(X, y[, sample_weight])Fit the calibrated model.
Get metadata routing of this object.
get_params
([deep])Get parameters for this estimator.
predict
(X)Predict the target of new samples.
Calibrated probabilities of classification.
score
(X, y[, sample_weight])Return the mean accuracy on the given test data and labels.
set_fit_request
(*[, sample_weight])Request metadata passed to the
fit
method.set_params
(**params)Set the parameters of this estimator.
set_score_request
(*[, sample_weight])Request metadata passed to the
score
method.- fit(X, y, sample_weight=None, **fit_params)[source]¶
Fit the calibrated model.
- Parameters:
- Xarray-like of shape (n_samples, n_features)
Training data.
- yarray-like of shape (n_samples,)
Target values.
- sample_weightarray-like of shape (n_samples,), default=None
Sample weights. If None, then samples are equally weighted.
- **fit_paramsdict
Parameters to pass to the
fit
method of the underlying classifier.
- Returns:
- selfobject
Returns an instance of self.
- get_metadata_routing()[source]¶
Get metadata routing of this object.
Please check User Guide on how the routing mechanism works.
- Returns:
- routingMetadataRouter
A
MetadataRouter
encapsulating routing information.
- get_params(deep=True)[source]¶
Get parameters for this estimator.
- Parameters:
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns:
- paramsdict
Parameter names mapped to their values.
- predict(X)[source]¶
Predict the target of new samples.
The predicted class is the class that has the highest probability, and can thus be different from the prediction of the uncalibrated classifier.
- Parameters:
- Xarray-like of shape (n_samples, n_features)
The samples, as accepted by
estimator.predict
.
- Returns:
- Cndarray of shape (n_samples,)
The predicted class.
- predict_proba(X)[source]¶
Calibrated probabilities of classification.
This function returns calibrated probabilities of classification according to each class on an array of test vectors X.
- Parameters:
- Xarray-like of shape (n_samples, n_features)
The samples, as accepted by
estimator.predict_proba
.
- Returns:
- Cndarray of shape (n_samples, n_classes)
The predicted probas.
- score(X, y, sample_weight=None)[source]¶
Return the mean accuracy on the given test data and labels.
In multi-label classification, this is the subset accuracy which is a harsh metric since you require for each sample that each label set be correctly predicted.
- Parameters:
- Xarray-like of shape (n_samples, n_features)
Test samples.
- yarray-like of shape (n_samples,) or (n_samples, n_outputs)
True labels for
X
.- sample_weightarray-like of shape (n_samples,), default=None
Sample weights.
- Returns:
- scorefloat
Mean accuracy of
self.predict(X)
w.r.t.y
.
- set_fit_request(*, sample_weight: bool | None | str = '$UNCHANGED$') CalibratedClassifierCV [source]¶
Request metadata passed to the
fit
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed tofit
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it tofit
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- sample_weightstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
sample_weight
parameter infit
.
- Returns:
- selfobject
The updated object.
- set_params(**params)[source]¶
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters:
- **paramsdict
Estimator parameters.
- Returns:
- selfestimator instance
Estimator instance.
- set_score_request(*, sample_weight: bool | None | str = '$UNCHANGED$') CalibratedClassifierCV [source]¶
Request metadata passed to the
score
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed toscore
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it toscore
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- sample_weightstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
sample_weight
parameter inscore
.
- Returns:
- selfobject
The updated object.
Examples using sklearn.calibration.CalibratedClassifierCV
¶
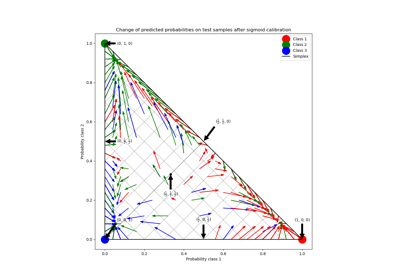
Probability Calibration for 3-class classification