Note
Click here to download the full example code
Selecting dimensionality reduction with Pipeline and GridSearchCV¶
This example constructs a pipeline that does dimensionality
reduction followed by prediction with a support vector
classifier. It demonstrates the use of GridSearchCV
and
Pipeline
to optimize over different classes of estimators in a
single CV run – unsupervised PCA
and NMF
dimensionality
reductions are compared to univariate feature selection during
the grid search.
Additionally, Pipeline
can be instantiated with the memory
argument to memoize the transformers within the pipeline, avoiding to fit
again the same transformers over and over.
Note that the use of memory
to enable caching becomes interesting when the
fitting of a transformer is costly.
Illustration of Pipeline
and GridSearchCV
¶
This section illustrates the use of aPipeline
withGridSearchCV
# Authors: Robert McGibbon, Joel Nothman, Guillaume Lemaitre
from __future__ import print_function, division
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_digits
from sklearn.model_selection import GridSearchCV
from sklearn.pipeline import Pipeline
from sklearn.svm import LinearSVC
from sklearn.decomposition import PCA, NMF
from sklearn.feature_selection import SelectKBest, chi2
print(__doc__)
pipe = Pipeline([
# the reduce_dim stage is populated by the param_grid
('reduce_dim', None),
('classify', LinearSVC())
])
N_FEATURES_OPTIONS = [2, 4, 8]
C_OPTIONS = [1, 10, 100, 1000]
param_grid = [
{
'reduce_dim': [PCA(iterated_power=7), NMF()],
'reduce_dim__n_components': N_FEATURES_OPTIONS,
'classify__C': C_OPTIONS
},
{
'reduce_dim': [SelectKBest(chi2)],
'reduce_dim__k': N_FEATURES_OPTIONS,
'classify__C': C_OPTIONS
},
]
reducer_labels = ['PCA', 'NMF', 'KBest(chi2)']
grid = GridSearchCV(pipe, cv=5, n_jobs=1, param_grid=param_grid)
digits = load_digits()
grid.fit(digits.data, digits.target)
mean_scores = np.array(grid.cv_results_['mean_test_score'])
# scores are in the order of param_grid iteration, which is alphabetical
mean_scores = mean_scores.reshape(len(C_OPTIONS), -1, len(N_FEATURES_OPTIONS))
# select score for best C
mean_scores = mean_scores.max(axis=0)
bar_offsets = (np.arange(len(N_FEATURES_OPTIONS)) *
(len(reducer_labels) + 1) + .5)
plt.figure()
COLORS = 'bgrcmyk'
for i, (label, reducer_scores) in enumerate(zip(reducer_labels, mean_scores)):
plt.bar(bar_offsets + i, reducer_scores, label=label, color=COLORS[i])
plt.title("Comparing feature reduction techniques")
plt.xlabel('Reduced number of features')
plt.xticks(bar_offsets + len(reducer_labels) / 2, N_FEATURES_OPTIONS)
plt.ylabel('Digit classification accuracy')
plt.ylim((0, 1))
plt.legend(loc='upper left')
plt.show()
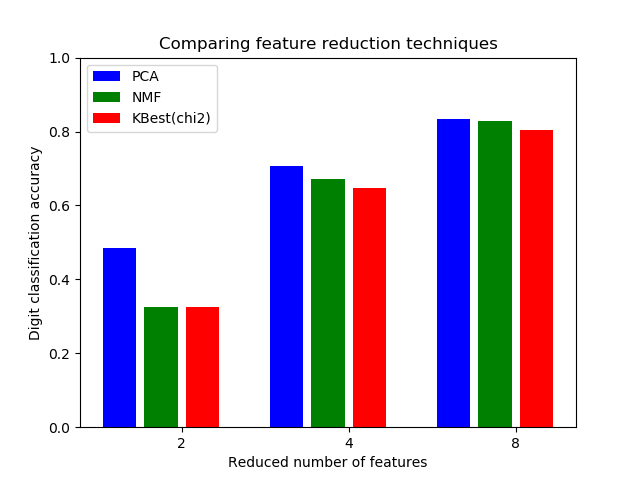
Out:
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/model_selection/_search.py:842: DeprecationWarning: The default of the `iid` parameter will change from True to False in version 0.22 and will be removed in 0.24. This will change numeric results when test-set sizes are unequal.
DeprecationWarning)
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
Caching transformers within a Pipeline
¶
It is sometimes worthwhile storing the state of a specific transformer since it could be used again. Using a pipeline in
GridSearchCV
triggers such situations. Therefore, we use the argumentmemory
to enable caching.Warning
Note that this example is, however, only an illustration since for this specific case fitting PCA is not necessarily slower than loading the cache. Hence, use the
memory
constructor parameter when the fitting of a transformer is costly.
from tempfile import mkdtemp
from shutil import rmtree
from joblib import Memory
# Create a temporary folder to store the transformers of the pipeline
cachedir = mkdtemp()
memory = Memory(cachedir=cachedir, verbose=10)
cached_pipe = Pipeline([('reduce_dim', PCA()),
('classify', LinearSVC())],
memory=memory)
# This time, a cached pipeline will be used within the grid search
grid = GridSearchCV(cached_pipe, cv=5, n_jobs=1, param_grid=param_grid)
digits = load_digits()
grid.fit(digits.data, digits.target)
# Delete the temporary cache before exiting
rmtree(cachedir)
Out:
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=2, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=2, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=2, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=2, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.2s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=2, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=4, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=4, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.2s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=4, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=4, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=4, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=8, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=8, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=8, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=8, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(copy=True, iterated_power=7, n_components=8, random_state=None,
svd_solver='auto', tol=0.0, whiten=False),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=2, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=2, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=2, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=2, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=2, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=4, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=4, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.2s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=4, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=4, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=4, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.2s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.2s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.3s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.3s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/99030190aa1cbbd235640b05063c22a1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd5af3545d6413292daf306fec7806e1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/6216faccde16e65b4babcfac673f3a3b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b39ee4c1313b78f35a274325259ea3b5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9cf54e3f15945d798d1439ae5056a296
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ccb071b592fd8b1f674776d43554c495
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/83da2ed5f842ca488aa1eba623501586
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/424887a3088180942118eba1a271e303
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/70f96c7d4e953424b10173451c0a188b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/91fab49bad8e01ee74bb30217740b852
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/837af6c3828cc968eaaf4732bb8ee772
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4f055cf1f6b4ff3a85402c9ca6a0f071
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/73a102a635a51fb132d4c7f1b7a59751
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/18d8ba25ab1b7e3804f24e1649e2d696
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/cce86d166377fa892602121608af4e8e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bb71d973d7e410f4abc584b1543385a0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bda6a969e2c8065ab5e3d0b17c1a9a37
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/21e5afee0b5eecdecb359617f48ff22d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a139bb2710313861c8514cf0d432837f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/5320bed678d7b056911eb23063fef569
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ada32f25a9da9a4da19f1ee6525e18a4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ce9b569be340e4927c7c80e076a75093
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd6bf3c8ff36e43d97a7c19a3182fd32
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ddb092001bbce9302f5f73046f5ebaf2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/8537805cc6e6e0c9c7a989cbce0cd4a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/e178166e1f4e48397c0c4dc766a57697
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/c35eaafc2193a546c2740027acd869c7
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d7f0d720f77cadfa170def63d597d157
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/3b5f3954049c5fbd4a8866c4a9efb628
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bcf3579286406ef213c40a928708eba6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/99030190aa1cbbd235640b05063c22a1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd5af3545d6413292daf306fec7806e1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/6216faccde16e65b4babcfac673f3a3b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b39ee4c1313b78f35a274325259ea3b5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9cf54e3f15945d798d1439ae5056a296
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ccb071b592fd8b1f674776d43554c495
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/83da2ed5f842ca488aa1eba623501586
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/424887a3088180942118eba1a271e303
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/70f96c7d4e953424b10173451c0a188b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/91fab49bad8e01ee74bb30217740b852
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/837af6c3828cc968eaaf4732bb8ee772
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4f055cf1f6b4ff3a85402c9ca6a0f071
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/73a102a635a51fb132d4c7f1b7a59751
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/18d8ba25ab1b7e3804f24e1649e2d696
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/cce86d166377fa892602121608af4e8e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bb71d973d7e410f4abc584b1543385a0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bda6a969e2c8065ab5e3d0b17c1a9a37
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/21e5afee0b5eecdecb359617f48ff22d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a139bb2710313861c8514cf0d432837f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/5320bed678d7b056911eb23063fef569
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ada32f25a9da9a4da19f1ee6525e18a4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ce9b569be340e4927c7c80e076a75093
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd6bf3c8ff36e43d97a7c19a3182fd32
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ddb092001bbce9302f5f73046f5ebaf2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/8537805cc6e6e0c9c7a989cbce0cd4a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/e178166e1f4e48397c0c4dc766a57697
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/c35eaafc2193a546c2740027acd869c7
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d7f0d720f77cadfa170def63d597d157
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/3b5f3954049c5fbd4a8866c4a9efb628
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bcf3579286406ef213c40a928708eba6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/99030190aa1cbbd235640b05063c22a1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd5af3545d6413292daf306fec7806e1
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/6216faccde16e65b4babcfac673f3a3b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b39ee4c1313b78f35a274325259ea3b5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9cf54e3f15945d798d1439ae5056a296
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ccb071b592fd8b1f674776d43554c495
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/83da2ed5f842ca488aa1eba623501586
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/424887a3088180942118eba1a271e303
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/70f96c7d4e953424b10173451c0a188b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/91fab49bad8e01ee74bb30217740b852
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/837af6c3828cc968eaaf4732bb8ee772
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4f055cf1f6b4ff3a85402c9ca6a0f071
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/73a102a635a51fb132d4c7f1b7a59751
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/18d8ba25ab1b7e3804f24e1649e2d696
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/cce86d166377fa892602121608af4e8e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bb71d973d7e410f4abc584b1543385a0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bda6a969e2c8065ab5e3d0b17c1a9a37
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/21e5afee0b5eecdecb359617f48ff22d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a139bb2710313861c8514cf0d432837f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/5320bed678d7b056911eb23063fef569
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ada32f25a9da9a4da19f1ee6525e18a4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ce9b569be340e4927c7c80e076a75093
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fd6bf3c8ff36e43d97a7c19a3182fd32
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ddb092001bbce9302f5f73046f5ebaf2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/8537805cc6e6e0c9c7a989cbce0cd4a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/e178166e1f4e48397c0c4dc766a57697
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/c35eaafc2193a546c2740027acd869c7
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d7f0d720f77cadfa170def63d597d157
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/3b5f3954049c5fbd4a8866c4a9efb628
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bcf3579286406ef213c40a928708eba6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7f3f22707ea0>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None)
________________________________________________fit_transform_one - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9f03feae786e954eb655cec568dbd930
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d97d229d8dabde9676fb389c893f790a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fbd4f8d0d4a89396347768eac1ab58e0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a4fd1cea85200e1f0083111dcb37e844
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b35adeadb235d13b6ec18f3807db6412
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a06623d6c70fa52487768058e2187806
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/38415c6fae02ab7e5d12ab8a29472e28
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fdb40226ed5f577ddfe2f8a21f79b5f5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ad90c157b42567e57efafe55e0fbbe73
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bbf3c60a42b256a682c9d61a8451efbc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4d587f8dbb456fd803125ba30f282a4a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/84d1c0d0b1ee5d7cc0684ffb6bd98984
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/eab5dca57bb193865942a8d3f01d45a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/619e5295cc89673aef757731c5243834
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/806aeb48cbe43bcb1b71cc03c7774b71
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9f03feae786e954eb655cec568dbd930
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d97d229d8dabde9676fb389c893f790a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fbd4f8d0d4a89396347768eac1ab58e0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a4fd1cea85200e1f0083111dcb37e844
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b35adeadb235d13b6ec18f3807db6412
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a06623d6c70fa52487768058e2187806
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/38415c6fae02ab7e5d12ab8a29472e28
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fdb40226ed5f577ddfe2f8a21f79b5f5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ad90c157b42567e57efafe55e0fbbe73
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bbf3c60a42b256a682c9d61a8451efbc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4d587f8dbb456fd803125ba30f282a4a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/84d1c0d0b1ee5d7cc0684ffb6bd98984
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/eab5dca57bb193865942a8d3f01d45a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/619e5295cc89673aef757731c5243834
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/806aeb48cbe43bcb1b71cc03c7774b71
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/9f03feae786e954eb655cec568dbd930
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/d97d229d8dabde9676fb389c893f790a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fbd4f8d0d4a89396347768eac1ab58e0
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a4fd1cea85200e1f0083111dcb37e844
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/b35adeadb235d13b6ec18f3807db6412
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/a06623d6c70fa52487768058e2187806
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/38415c6fae02ab7e5d12ab8a29472e28
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/fdb40226ed5f577ddfe2f8a21f79b5f5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/ad90c157b42567e57efafe55e0fbbe73
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/bbf3c60a42b256a682c9d61a8451efbc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/4d587f8dbb456fd803125ba30f282a4a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/84d1c0d0b1ee5d7cc0684ffb6bd98984
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/eab5dca57bb193865942a8d3f01d45a9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/619e5295cc89673aef757731c5243834
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmp7mq43s6y/joblib/sklearn/pipeline/_fit_transform_one/806aeb48cbe43bcb1b71cc03c7774b71
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
/home/circleci/project/sklearn/svm/base.py:931: ConvergenceWarning: Liblinear failed to converge, increase the number of iterations.
"the number of iterations.", ConvergenceWarning)
/home/circleci/project/sklearn/model_selection/_search.py:842: DeprecationWarning: The default of the `iid` parameter will change from True to False in version 0.22 and will be removed in 0.24. This will change numeric results when test-set sizes are unequal.
DeprecationWarning)
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(alpha=0.0, beta_loss='frobenius', init=None, l1_ratio=0.0, max_iter=200,
n_components=8, random_state=None, shuffle=False, solver='cd',
tol=0.0001, verbose=0),
array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None)
________________________________________________fit_transform_one - 0.5s, 0.0min
The PCA
fitting is only computed at the evaluation of the first
configuration of the C
parameter of the LinearSVC
classifier. The
other configurations of C
will trigger the loading of the cached PCA
estimator data, leading to save processing time. Therefore, the use of
caching the pipeline using memory
is highly beneficial when fitting
a transformer is costly.
Total running time of the script: ( 2 minutes 45.328 seconds)