sklearn.feature_selection
.RFECV¶
- class sklearn.feature_selection.RFECV(estimator, *, step=1, min_features_to_select=1, cv=None, scoring=None, verbose=0, n_jobs=None, importance_getter='auto')[source]¶
Recursive feature elimination with cross-validation to select features.
The number of features selected is tuned automatically by fitting an
RFE
selector on the different cross-validation splits (provided by thecv
parameter). The performance of theRFE
selector are evaluated usingscorer
for different number of selected features and aggregated together. Finally, the scores are averaged across folds and the number of features selected is set to the number of features that maximize the cross-validation score. See glossary entry for cross-validation estimator.Read more in the User Guide.
- Parameters:
- estimator
Estimator
instance A supervised learning estimator with a
fit
method that provides information about feature importance either through acoef_
attribute or through afeature_importances_
attribute.- stepint or float, default=1
If greater than or equal to 1, then
step
corresponds to the (integer) number of features to remove at each iteration. If within (0.0, 1.0), thenstep
corresponds to the percentage (rounded down) of features to remove at each iteration. Note that the last iteration may remove fewer thanstep
features in order to reachmin_features_to_select
.- min_features_to_selectint, default=1
The minimum number of features to be selected. This number of features will always be scored, even if the difference between the original feature count and
min_features_to_select
isn’t divisible bystep
.New in version 0.20.
- cvint, cross-validation generator or an iterable, default=None
Determines the cross-validation splitting strategy. Possible inputs for cv are:
None, to use the default 5-fold cross-validation,
integer, to specify the number of folds.
An iterable yielding (train, test) splits as arrays of indices.
For integer/None inputs, if
y
is binary or multiclass,StratifiedKFold
is used. If the estimator is a classifier or ify
is neither binary nor multiclass,KFold
is used.Refer User Guide for the various cross-validation strategies that can be used here.
Changed in version 0.22:
cv
default value of None changed from 3-fold to 5-fold.- scoringstr, callable or None, default=None
A string (see model evaluation documentation) or a scorer callable object / function with signature
scorer(estimator, X, y)
.- verboseint, default=0
Controls verbosity of output.
- n_jobsint or None, default=None
Number of cores to run in parallel while fitting across folds.
None
means 1 unless in ajoblib.parallel_backend
context.-1
means using all processors. See Glossary for more details.New in version 0.18.
- importance_getterstr or callable, default=’auto’
If ‘auto’, uses the feature importance either through a
coef_
orfeature_importances_
attributes of estimator.Also accepts a string that specifies an attribute name/path for extracting feature importance. For example, give
regressor_.coef_
in case ofTransformedTargetRegressor
ornamed_steps.clf.feature_importances_
in case ofPipeline
with its last step namedclf
.If
callable
, overrides the default feature importance getter. The callable is passed with the fitted estimator and it should return importance for each feature.New in version 0.24.
- estimator
- Attributes:
classes_
ndarray of shape (n_classes,)Classes labels available when
estimator
is a classifier.- estimator_
Estimator
instance The fitted estimator used to select features.
- cv_results_dict of ndarrays
A dict with keys:
- split(k)_test_scorendarray of shape (n_subsets_of_features,)
The cross-validation scores across (k)th fold.
- mean_test_scorendarray of shape (n_subsets_of_features,)
Mean of scores over the folds.
- std_test_scorendarray of shape (n_subsets_of_features,)
Standard deviation of scores over the folds.
New in version 1.0.
- n_features_int
The number of selected features with cross-validation.
- n_features_in_int
Number of features seen during fit. Only defined if the underlying estimator exposes such an attribute when fit.
New in version 0.24.
- feature_names_in_ndarray of shape (
n_features_in_
,) Names of features seen during fit. Defined only when
X
has feature names that are all strings.New in version 1.0.
- ranking_narray of shape (n_features,)
The feature ranking, such that
ranking_[i]
corresponds to the ranking position of the i-th feature. Selected (i.e., estimated best) features are assigned rank 1.- support_ndarray of shape (n_features,)
The mask of selected features.
See also
RFE
Recursive feature elimination.
Notes
The size of all values in
cv_results_
is equal toceil((n_features - min_features_to_select) / step) + 1
, where step is the number of features removed at each iteration.Allows NaN/Inf in the input if the underlying estimator does as well.
References
[1]Guyon, I., Weston, J., Barnhill, S., & Vapnik, V., “Gene selection for cancer classification using support vector machines”, Mach. Learn., 46(1-3), 389–422, 2002.
Examples
The following example shows how to retrieve the a-priori not known 5 informative features in the Friedman #1 dataset.
>>> from sklearn.datasets import make_friedman1 >>> from sklearn.feature_selection import RFECV >>> from sklearn.svm import SVR >>> X, y = make_friedman1(n_samples=50, n_features=10, random_state=0) >>> estimator = SVR(kernel="linear") >>> selector = RFECV(estimator, step=1, cv=5) >>> selector = selector.fit(X, y) >>> selector.support_ array([ True, True, True, True, True, False, False, False, False, False]) >>> selector.ranking_ array([1, 1, 1, 1, 1, 6, 4, 3, 2, 5])
Methods
Compute the decision function of
X
.fit
(X, y[, groups])Fit the RFE model and automatically tune the number of selected features.
fit_transform
(X[, y])Fit to data, then transform it.
get_feature_names_out
([input_features])Mask feature names according to selected features.
Raise
NotImplementedError
.get_params
([deep])Get parameters for this estimator.
get_support
([indices])Get a mask, or integer index, of the features selected.
Reverse the transformation operation.
predict
(X)Reduce X to the selected features and predict using the estimator.
Predict class log-probabilities for X.
Predict class probabilities for X.
score
(X, y, **fit_params)Reduce X to the selected features and return the score of the estimator.
set_fit_request
(*[, groups])Request metadata passed to the
fit
method.set_output
(*[, transform])Set output container.
set_params
(**params)Set the parameters of this estimator.
transform
(X)Reduce X to the selected features.
- property classes_¶
Classes labels available when
estimator
is a classifier.- Returns:
- ndarray of shape (n_classes,)
- decision_function(X)[source]¶
Compute the decision function of
X
.- Parameters:
- X{array-like or sparse matrix} of shape (n_samples, n_features)
The input samples. Internally, it will be converted to
dtype=np.float32
and if a sparse matrix is provided to a sparsecsr_matrix
.
- Returns:
- scorearray, shape = [n_samples, n_classes] or [n_samples]
The decision function of the input samples. The order of the classes corresponds to that in the attribute classes_. Regression and binary classification produce an array of shape [n_samples].
- fit(X, y, groups=None)[source]¶
Fit the RFE model and automatically tune the number of selected features.
- Parameters:
- X{array-like, sparse matrix} of shape (n_samples, n_features)
Training vector, where
n_samples
is the number of samples andn_features
is the total number of features.- yarray-like of shape (n_samples,)
Target values (integers for classification, real numbers for regression).
- groupsarray-like of shape (n_samples,) or None, default=None
Group labels for the samples used while splitting the dataset into train/test set. Only used in conjunction with a “Group” cv instance (e.g.,
GroupKFold
).New in version 0.20.
- Returns:
- selfobject
Fitted estimator.
- fit_transform(X, y=None, **fit_params)[source]¶
Fit to data, then transform it.
Fits transformer to
X
andy
with optional parametersfit_params
and returns a transformed version ofX
.- Parameters:
- Xarray-like of shape (n_samples, n_features)
Input samples.
- yarray-like of shape (n_samples,) or (n_samples, n_outputs), default=None
Target values (None for unsupervised transformations).
- **fit_paramsdict
Additional fit parameters.
- Returns:
- X_newndarray array of shape (n_samples, n_features_new)
Transformed array.
- get_feature_names_out(input_features=None)[source]¶
Mask feature names according to selected features.
- Parameters:
- input_featuresarray-like of str or None, default=None
Input features.
If
input_features
isNone
, thenfeature_names_in_
is used as feature names in. Iffeature_names_in_
is not defined, then the following input feature names are generated:["x0", "x1", ..., "x(n_features_in_ - 1)"]
.If
input_features
is an array-like, theninput_features
must matchfeature_names_in_
iffeature_names_in_
is defined.
- Returns:
- feature_names_outndarray of str objects
Transformed feature names.
- get_metadata_routing()[source]¶
Raise
NotImplementedError
.This estimator does not support metadata routing yet.
- get_params(deep=True)[source]¶
Get parameters for this estimator.
- Parameters:
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns:
- paramsdict
Parameter names mapped to their values.
- get_support(indices=False)[source]¶
Get a mask, or integer index, of the features selected.
- Parameters:
- indicesbool, default=False
If True, the return value will be an array of integers, rather than a boolean mask.
- Returns:
- supportarray
An index that selects the retained features from a feature vector. If
indices
is False, this is a boolean array of shape [# input features], in which an element is True iff its corresponding feature is selected for retention. Ifindices
is True, this is an integer array of shape [# output features] whose values are indices into the input feature vector.
- inverse_transform(X)[source]¶
Reverse the transformation operation.
- Parameters:
- Xarray of shape [n_samples, n_selected_features]
The input samples.
- Returns:
- X_rarray of shape [n_samples, n_original_features]
X
with columns of zeros inserted where features would have been removed bytransform
.
- predict(X)[source]¶
Reduce X to the selected features and predict using the estimator.
- Parameters:
- Xarray of shape [n_samples, n_features]
The input samples.
- Returns:
- yarray of shape [n_samples]
The predicted target values.
- predict_log_proba(X)[source]¶
Predict class log-probabilities for X.
- Parameters:
- Xarray of shape [n_samples, n_features]
The input samples.
- Returns:
- parray of shape (n_samples, n_classes)
The class log-probabilities of the input samples. The order of the classes corresponds to that in the attribute classes_.
- predict_proba(X)[source]¶
Predict class probabilities for X.
- Parameters:
- X{array-like or sparse matrix} of shape (n_samples, n_features)
The input samples. Internally, it will be converted to
dtype=np.float32
and if a sparse matrix is provided to a sparsecsr_matrix
.
- Returns:
- parray of shape (n_samples, n_classes)
The class probabilities of the input samples. The order of the classes corresponds to that in the attribute classes_.
- score(X, y, **fit_params)[source]¶
Reduce X to the selected features and return the score of the estimator.
- Parameters:
- Xarray of shape [n_samples, n_features]
The input samples.
- yarray of shape [n_samples]
The target values.
- **fit_paramsdict
Parameters to pass to the
score
method of the underlying estimator.New in version 1.0.
- Returns:
- scorefloat
Score of the underlying base estimator computed with the selected features returned by
rfe.transform(X)
andy
.
- set_fit_request(*, groups: bool | None | str = '$UNCHANGED$') RFECV [source]¶
Request metadata passed to the
fit
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed tofit
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it tofit
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- groupsstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
groups
parameter infit
.
- Returns:
- selfobject
The updated object.
- set_output(*, transform=None)[source]¶
Set output container.
See Introducing the set_output API for an example on how to use the API.
- Parameters:
- transform{“default”, “pandas”}, default=None
Configure output of
transform
andfit_transform
."default"
: Default output format of a transformer"pandas"
: DataFrame output"polars"
: Polars outputNone
: Transform configuration is unchanged
New in version 1.4:
"polars"
option was added.
- Returns:
- selfestimator instance
Estimator instance.
- set_params(**params)[source]¶
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters:
- **paramsdict
Estimator parameters.
- Returns:
- selfestimator instance
Estimator instance.
Examples using sklearn.feature_selection.RFECV
¶
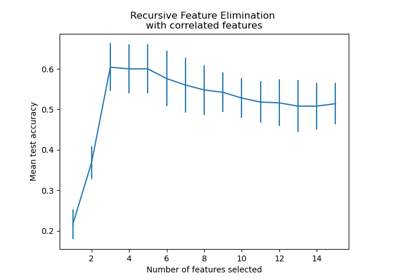
Recursive feature elimination with cross-validation