Note
Click here to download the full example code
Selecting dimensionality reduction with Pipeline and GridSearchCV¶
This example constructs a pipeline that does dimensionality
reduction followed by prediction with a support vector
classifier. It demonstrates the use of GridSearchCV
and
Pipeline
to optimize over different classes of estimators in a
single CV run – unsupervised PCA
and NMF
dimensionality
reductions are compared to univariate feature selection during
the grid search.
Additionally, Pipeline
can be instantiated with the memory
argument to memoize the transformers within the pipeline, avoiding to fit
again the same transformers over and over.
Note that the use of memory
to enable caching becomes interesting when the
fitting of a transformer is costly.
Illustration of Pipeline
and GridSearchCV
¶
This section illustrates the use of aPipeline
withGridSearchCV
# Authors: Robert McGibbon, Joel Nothman, Guillaume Lemaitre
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_digits
from sklearn.model_selection import GridSearchCV
from sklearn.pipeline import Pipeline
from sklearn.svm import LinearSVC
from sklearn.decomposition import PCA, NMF
from sklearn.feature_selection import SelectKBest, chi2
print(__doc__)
pipe = Pipeline([
# the reduce_dim stage is populated by the param_grid
('reduce_dim', 'passthrough'),
('classify', LinearSVC(dual=False, max_iter=10000))
])
N_FEATURES_OPTIONS = [2, 4, 8]
C_OPTIONS = [1, 10, 100, 1000]
param_grid = [
{
'reduce_dim': [PCA(iterated_power=7), NMF()],
'reduce_dim__n_components': N_FEATURES_OPTIONS,
'classify__C': C_OPTIONS
},
{
'reduce_dim': [SelectKBest(chi2)],
'reduce_dim__k': N_FEATURES_OPTIONS,
'classify__C': C_OPTIONS
},
]
reducer_labels = ['PCA', 'NMF', 'KBest(chi2)']
grid = GridSearchCV(pipe, cv=5, n_jobs=1, param_grid=param_grid, iid=False)
digits = load_digits()
grid.fit(digits.data, digits.target)
mean_scores = np.array(grid.cv_results_['mean_test_score'])
# scores are in the order of param_grid iteration, which is alphabetical
mean_scores = mean_scores.reshape(len(C_OPTIONS), -1, len(N_FEATURES_OPTIONS))
# select score for best C
mean_scores = mean_scores.max(axis=0)
bar_offsets = (np.arange(len(N_FEATURES_OPTIONS)) *
(len(reducer_labels) + 1) + .5)
plt.figure()
COLORS = 'bgrcmyk'
for i, (label, reducer_scores) in enumerate(zip(reducer_labels, mean_scores)):
plt.bar(bar_offsets + i, reducer_scores, label=label, color=COLORS[i])
plt.title("Comparing feature reduction techniques")
plt.xlabel('Reduced number of features')
plt.xticks(bar_offsets + len(reducer_labels) / 2, N_FEATURES_OPTIONS)
plt.ylabel('Digit classification accuracy')
plt.ylim((0, 1))
plt.legend(loc='upper left')
plt.show()
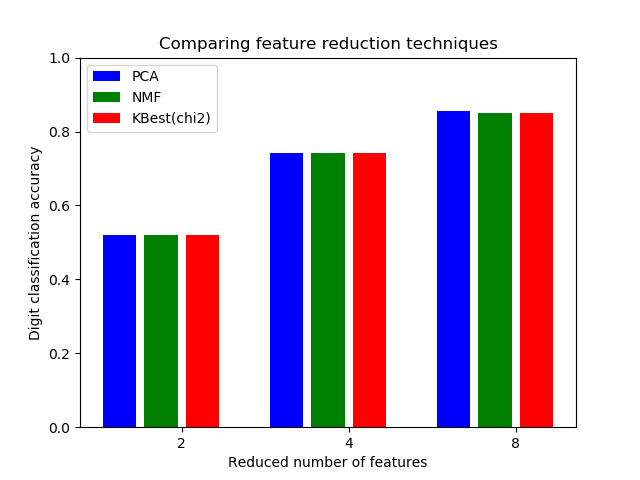
Caching transformers within a Pipeline
¶
It is sometimes worthwhile storing the state of a specific transformer since it could be used again. Using a pipeline in
GridSearchCV
triggers such situations. Therefore, we use the argumentmemory
to enable caching.Warning
Note that this example is, however, only an illustration since for this specific case fitting PCA is not necessarily slower than loading the cache. Hence, use the
memory
constructor parameter when the fitting of a transformer is costly.
from tempfile import mkdtemp
from shutil import rmtree
from joblib import Memory
# Create a temporary folder to store the transformers of the pipeline
cachedir = mkdtemp()
memory = Memory(location=cachedir, verbose=10)
cached_pipe = Pipeline([('reduce_dim', PCA()),
('classify', LinearSVC(dual=False, max_iter=10000))],
memory=memory)
# This time, a cached pipeline will be used within the grid search
grid = GridSearchCV(cached_pipe, cv=5, n_jobs=1, param_grid=param_grid,
iid=False)
digits = load_digits()
grid.fit(digits.data, digits.target)
# Delete the temporary cache before exiting
rmtree(cachedir)
Out:
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=2), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=4), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.2s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(NMF(n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.1s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/591acae8e60c9632aa990e4fa0962a67
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/323909961fcc2a4950defb581f08007f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3654e1d61587aee4f9980c99541d55d3
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/835820e75be3172b4e2e257028147bb2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/90604ce8bb756e3ff1acd4c1ce065b1a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3c66df347f488dfe044ecf991ca2498b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/bf4bec5fe5245bf3c0d271e31bc2342f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ce0f911df93a8e38932c48f983e7fde6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/285efba3f9e5b1a35d6825e37a718019
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/74083d6e1ed743fa1e756a41ba467e6c
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b72f745822a406f2191b6ebfd8ca9df9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/997e4ce30ba5143e90330db664bf61c5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3140177f6ccbe72992772342347a166f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f843896ad42a20c4a50c0fd29512111a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d91a2d083b8c7311c87edd6592fcd446
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/50eb0f4152cd0f7dce3b471add31d07d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3b28284b39c934cb519f2a31e03a951b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/aded0eb932b9c692c801c6c56dc2bda2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/cdf3d1530a8a2388ba80a16c66413409
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ff5666a0cb943711a4e4dfd5b5cd8587
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/536a5fbb5a6c8d69b4fb426f0b51d9eb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/26cd3a8242acb986f98cf38291ef5baa
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/8b6094f421dab9fc88fb54c2f32e16b4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d0e8598c62dcb5f059a5c2b541c23b09
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/34affb50a3d55c5302e1c7f97aec9679
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b3635522e8f89bbe9e56ab7e7f4693fc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7389ad9bae0ad1181b7da9e4dd39449d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ab59173e00ab4a7bdf7740e5c6d50123
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/42894538fd0ab2b4235e55ef53b9cd59
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/580596a10617556e39d5e069c4de096a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/591acae8e60c9632aa990e4fa0962a67
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/323909961fcc2a4950defb581f08007f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3654e1d61587aee4f9980c99541d55d3
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/835820e75be3172b4e2e257028147bb2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/90604ce8bb756e3ff1acd4c1ce065b1a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3c66df347f488dfe044ecf991ca2498b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/bf4bec5fe5245bf3c0d271e31bc2342f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ce0f911df93a8e38932c48f983e7fde6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/285efba3f9e5b1a35d6825e37a718019
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/74083d6e1ed743fa1e756a41ba467e6c
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b72f745822a406f2191b6ebfd8ca9df9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/997e4ce30ba5143e90330db664bf61c5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3140177f6ccbe72992772342347a166f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f843896ad42a20c4a50c0fd29512111a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d91a2d083b8c7311c87edd6592fcd446
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/50eb0f4152cd0f7dce3b471add31d07d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3b28284b39c934cb519f2a31e03a951b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/aded0eb932b9c692c801c6c56dc2bda2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/cdf3d1530a8a2388ba80a16c66413409
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ff5666a0cb943711a4e4dfd5b5cd8587
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/536a5fbb5a6c8d69b4fb426f0b51d9eb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/26cd3a8242acb986f98cf38291ef5baa
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/8b6094f421dab9fc88fb54c2f32e16b4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d0e8598c62dcb5f059a5c2b541c23b09
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/34affb50a3d55c5302e1c7f97aec9679
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b3635522e8f89bbe9e56ab7e7f4693fc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7389ad9bae0ad1181b7da9e4dd39449d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ab59173e00ab4a7bdf7740e5c6d50123
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/42894538fd0ab2b4235e55ef53b9cd59
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/580596a10617556e39d5e069c4de096a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/591acae8e60c9632aa990e4fa0962a67
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/323909961fcc2a4950defb581f08007f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3654e1d61587aee4f9980c99541d55d3
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/835820e75be3172b4e2e257028147bb2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/90604ce8bb756e3ff1acd4c1ce065b1a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3c66df347f488dfe044ecf991ca2498b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/bf4bec5fe5245bf3c0d271e31bc2342f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ce0f911df93a8e38932c48f983e7fde6
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/285efba3f9e5b1a35d6825e37a718019
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/74083d6e1ed743fa1e756a41ba467e6c
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b72f745822a406f2191b6ebfd8ca9df9
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/997e4ce30ba5143e90330db664bf61c5
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3140177f6ccbe72992772342347a166f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f843896ad42a20c4a50c0fd29512111a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d91a2d083b8c7311c87edd6592fcd446
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/50eb0f4152cd0f7dce3b471add31d07d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/3b28284b39c934cb519f2a31e03a951b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/aded0eb932b9c692c801c6c56dc2bda2
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/cdf3d1530a8a2388ba80a16c66413409
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ff5666a0cb943711a4e4dfd5b5cd8587
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/536a5fbb5a6c8d69b4fb426f0b51d9eb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/26cd3a8242acb986f98cf38291ef5baa
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/8b6094f421dab9fc88fb54c2f32e16b4
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d0e8598c62dcb5f059a5c2b541c23b09
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/34affb50a3d55c5302e1c7f97aec9679
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/b3635522e8f89bbe9e56ab7e7f4693fc
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7389ad9bae0ad1181b7da9e4dd39449d
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/ab59173e00ab4a7bdf7740e5c6d50123
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/42894538fd0ab2b4235e55ef53b9cd59
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/580596a10617556e39d5e069c4de096a
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=2, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=4, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(SelectKBest(k=8, score_func=<function chi2 at 0x7efe30bb2268>), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 9]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/0c252f8c2af87fe4a595ca853ea983cd
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/5feb279acc70b729ede7514b133d0172
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/6742bad9e26dd16b831fc9abd1883c9e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/096380a7ad90487199a4a6e8ec8a5744
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/e5107ef0d6468f91a5cd772a596ba876
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/2efa56788b791becec2fcd015ecf751f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d27c6056fc9ab1f3fb4327fd17346312
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d649c35672f6e2731789f5c6a9b03066
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/4906c4afa619398fb77288e086d5dd82
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7dc3c29273fd5c57e2913f82c9185294
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/63c1c1af7115994b5cd17a1189691d5b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/670746700924677f7a550fa4cae5c9bb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f9617292c7b763d23b3f6c9d96697c29
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/9a2bcb4639d1b192e5525c2bf0dca317
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/87c7c41885d63769a203e26b244fd823
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/0c252f8c2af87fe4a595ca853ea983cd
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/5feb279acc70b729ede7514b133d0172
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/6742bad9e26dd16b831fc9abd1883c9e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/096380a7ad90487199a4a6e8ec8a5744
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/e5107ef0d6468f91a5cd772a596ba876
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/2efa56788b791becec2fcd015ecf751f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d27c6056fc9ab1f3fb4327fd17346312
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d649c35672f6e2731789f5c6a9b03066
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/4906c4afa619398fb77288e086d5dd82
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7dc3c29273fd5c57e2913f82c9185294
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/63c1c1af7115994b5cd17a1189691d5b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/670746700924677f7a550fa4cae5c9bb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f9617292c7b763d23b3f6c9d96697c29
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/9a2bcb4639d1b192e5525c2bf0dca317
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/87c7c41885d63769a203e26b244fd823
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/0c252f8c2af87fe4a595ca853ea983cd
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/5feb279acc70b729ede7514b133d0172
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/6742bad9e26dd16b831fc9abd1883c9e
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/096380a7ad90487199a4a6e8ec8a5744
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/e5107ef0d6468f91a5cd772a596ba876
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/2efa56788b791becec2fcd015ecf751f
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d27c6056fc9ab1f3fb4327fd17346312
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/d649c35672f6e2731789f5c6a9b03066
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/4906c4afa619398fb77288e086d5dd82
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/7dc3c29273fd5c57e2913f82c9185294
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/63c1c1af7115994b5cd17a1189691d5b
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/670746700924677f7a550fa4cae5c9bb
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/f9617292c7b763d23b3f6c9d96697c29
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/9a2bcb4639d1b192e5525c2bf0dca317
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
[Memory]0.0s, 0.0min : Loading _fit_transform_one from /tmp/tmpl5ribdvu/joblib/sklearn/pipeline/_fit_transform_one/87c7c41885d63769a203e26b244fd823
___________________________________fit_transform_one cache loaded - 0.0s, 0.0min
________________________________________________________________________________
[Memory] Calling sklearn.pipeline._fit_transform_one...
_fit_transform_one(PCA(iterated_power=7, n_components=8), array([[0., ..., 0.],
...,
[0., ..., 0.]]), array([0, ..., 8]), None, message_clsname='Pipeline', message=None)
________________________________________________fit_transform_one - 0.0s, 0.0min
The PCA
fitting is only computed at the evaluation of the first
configuration of the C
parameter of the LinearSVC
classifier. The
other configurations of C
will trigger the loading of the cached PCA
estimator data, leading to save processing time. Therefore, the use of
caching the pipeline using memory
is highly beneficial when fitting
a transformer is costly.
Total running time of the script: ( 0 minutes 20.695 seconds)